Learning never stops especially when it comes to web development, knowledge is never enough. Every single day I see myself learning new things. If you think that getting a degree in computer science or taking that famous web development Bootcamp will make you a pro in the field then my friend you are wrong.
While doing any new side project I always end up creating something new that I had no prior knowledge of. This I feel is the fastest way of learning and gives me a lot of confidence.
Just like this, one day while working on a project I was looking for a pre-built forward arrow feature online. I was unable to find it so ended up coding one for myself. While looking for an online service for creating simple animations to formulating one in less than 1 hour for myself that fitted the requirement of my project, gave me an adrenaline rush.
I am super excited to share with you how I created the animated forward arrows feature with CSS3 animation keyframes. It’s simple to create and use in your website’s design.
Happy Learning!
Project
Here’s what you’ll be making by the end of this tutorial:
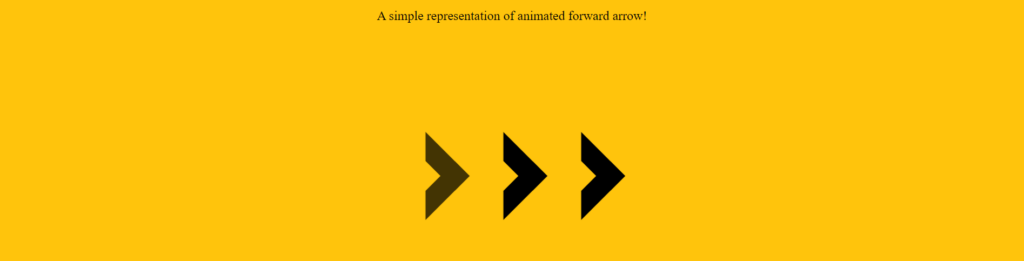
HTML
First, let’s write the basic HTML for this project.
<!-- HTML Code for Animated Forward Arrows -->
<p>A simple representation of animated forward arrow!</p>
<div id="ForwardArrow">
<div class="GlidingArrow">
<div class="arrow"></div>
</div>
<div class="GlidingArrow delay1">
<div class="arrow"></div>
</div>
<div class="GlidingArrow delay2">
<div class="arrow"></div>
</div>
<div class="GlidingArrow delay3">
<div class="arrow"></div>
</div>
</div>
I added a div
with the id
called ForwardArrow
. This div
is responsible for creating all the forward arrows elements. Inside this div id
, I added four div classes
with another class of arrow inside the main div class
elements, each representing a single arrow.
CSS Styles
Let’s first style our basic elements. The below code block defines the optional CSS styles for the p
tag and the body
to make your final project appealing to the eyes.
/* OPTIONAL: Styles for the demo. */
body {
background: #ffc40c;
padding: 2rem;
}
p {
font: 1rem/1.45 "Operator Mono";
color: black;
text-align: center;
}
Styling #ForwardArrow Id
Next, I styled my arrows with the following properties:
/* CSS for animated Forward Arrows.*/
#ForwardArrow {
width: 100vw;
height: 100vh;
display: flex;
justify-content: center;
align-items: center;
}
I used the flexbox (i.e., display: flex;
) property to place the animated forward arrows in the middle of the page both horizontally and vertically.
.arrow {
width: 2vw;
height: 2vw;
border: 2vw solid;
border-color: black transparent transparent black;
transform: rotate(-225deg);
}
.GlidingArrow {
position: absolute;
-webkit-animation: slide 5s linear infinite;
animation: slide 5s linear infinite;
}
.delay1 {
-webkit-animation-delay: 2s;
animation-delay: 2s;
}
.delay2 {
-webkit-animation-delay: 3s;
animation-delay: 3s;
}
.delay3 {
-webkit-animation-delay: 4s;
animation-delay: 4s;
}
To create an arrow I have given a constant viewport width i.e 2 vw for width, height, and border in the .arrow
class. The transform property rotated the arrow in the direction I wanted. You can play around and see what happens when you change the values.
The most interesting part here is the animation
property. I am using WebKit animation property to create multiple animations with prescribed delays. The first arrow runs once in 2s and repeats itself infinitely, the third after 3s and so. I am using this delay property so that each element does not start to animate at the same time.
Creating Animation Keyframe
Keyframes are used to define the animation behavior and give us complete control of one cycle of a CSS animation. To create an animation, I used the -webkit-animation
in conjunction with the @-webkit-keyframes
keyword/at-rule, which allowed me to define visual effects in my animation.
If you want several animation transitions, you can define a range of percentages each containing a list of styling selectors. That’s what I did inside the @keyframe.
These percentages can be listed in any order and with any difference between them. A simple representation of these percentages is shown below.
Let’s now write the code for the keyframe which I wrote to create my Forward Arrows Animation:
@-webkit-keyframes slide {
0% { opacity:0; transform: translateX(-15vw); }
20% { opacity:1; transform: translateX(-9vw); }
80% { opacity:1; transform: translateX(+9vw); }
100% { opacity:0; transform: translateX(+15vw); }
}
@keyframes slide {
0% { opacity:0; transform: translateX(-15vw); }
20% { opacity:1; transform: translateX(-9vw); }
80% { opacity:1; transform: translateX(+9vw); }
100% { opacity:0; transform: translateX(+15vw); }
}
To create the moving effect, I used the CSS transform
property to change the coordinates of a given element hence transforming the location of each arrow.
With this transform
property, I’ve used the translateX()
function which takes one input repositioning an element horizontally on the 2D plane.
The Final Product
Here’s the complete code of this animated Forward Arrows:
HTML Code
<!-- HTML Code for Animated Forward Arrows -->
<p>A simple representation of animated forward arrow!</p>
<div id="ForwardArrow">
<div class="GlidingArrow">
<div class="arrow"></div>
</div>
<div class="GlidingArrow delay1">
<div class="arrow"></div>
</div>
<div class="GlidingArrow delay2">
<div class="arrow"></div>
</div>
<div class="GlidingArrow delay3">
<div class="arrow"></div>
</div>
</div>
CSS Code
/* OPTIONAL: Styles for the demo. */
body {
background: #ffc40c;
padding: 2rem;
}
p {
font: 1rem/1.45 "Operator Mono";
color: black;
text-align: center;
}
/* CSS for animated Forward Arrows.*/
#ForwardArrow {
width: 100vw;
height: 100vh;
display: flex;
justify-content: center;
align-items: center;
}
.arrow {
width: 2vw;
height: 2vw;
border: 2vw solid;
border-color: black transparent transparent black;
transform: rotate(-225deg);
}
.GlidingArrow {
position: absolute;
-webkit-animation: slide 5s linear infinite;
animation: slide 5s linear infinite;
}
.delay1 {
-webkit-animation-delay: 2s;
animation-delay: 2s;
}
.delay2 {
-webkit-animation-delay: 3s;
animation-delay: 3s;
}
.delay3 {
-webkit-animation-delay: 4s;
animation-delay: 4s;
}
/* Defining animation Keyframes */
@-webkit-keyframes slide {
0% { opacity:0; transform: translateX(-15vw); }
20% { opacity:1; transform: translateX(-9vw); }
80% { opacity:1; transform: translateX(+9vw); }
100% { opacity:0; transform: translateX(+15vw); }
}
@keyframes slide {
0% { opacity:0; transform: translateX(-15vw); }
20% { opacity:1; transform: translateX(-9vw); }
80% { opacity:1; transform: translateX(+9vw); }
100% { opacity:0; transform: translateX(+15vw); }
}
Wrapping Up!
After developing this animation, I have realized that CSS is amazingly powerful. There are several ways of creating animations like this. I’d love to hear your suggestions and the way you create CSS animations.
Thanks for reading! If this tutorial was helpful try it out yourself and share your feedback in the comments section below.
Leave a Reply